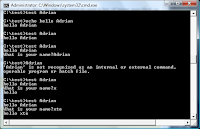
Batch files are useful for writing small automated scripts in windows.
You just type any command you like into a text-file with the extension ".bat" and it will do the magic for you.
Some extra commands/parameters will help:
%1,%2,%3 ... will let you pass additional paramters
echo will print something to the screen
@echo off will stop the bat file from printing everything to the screen
REM will make a line into a comment
> will let you pipe the output between commands, just like on *nix.
set /p variable= [string] will let you prompt for input
exist will let you test if a file exists
You also have programming constructs including 'if' and 'goto'. (For an example see this batch file sorting routine)
That's pretty much all you could ever need.
So a simple example, named test.bat:
@echo off
echo hello %1
If I call this with "test Adrian" it will print "hello Adrian" to the screen.
@echo off
set /p name= What is your name?
echo hello %name%
This does the same, except prompts me to enter my name.
There are more than just the %1..%n variable for commands, there is also %0 to tell you the name of the bat file. Even better, you can extract the path with %dp0
eg:
@echo off
echo %~dp0
So now you can invoke Tortoise SVN on the command line to download and install your favourite software.
For example:
tortoiseproc /command:checkout /path:%~dp0 pal /url:https://pal.svn.sourceforge.net/svnroot/pal/pal /closeonend:1
tortoiseproc /command:checkout /path:%~dp0 bullet /url:http://bullet.googlecode.com/svn/trunk /closeonend:1
Even better, we can extend this with exists to see if the code is already there, and do an update instead:
if not exist pal\NUL goto nopal
tortoiseproc /command:update /path:%~dp0pal /closeonend:1
goto NEXT
:nopal
tortoiseproc /command:checkout /path:%~dp0 pal /url:https://pal.svn.sourceforge.net/svnroot/pal/pal /closeonend:1
Enjoy!
No comments:
Post a Comment